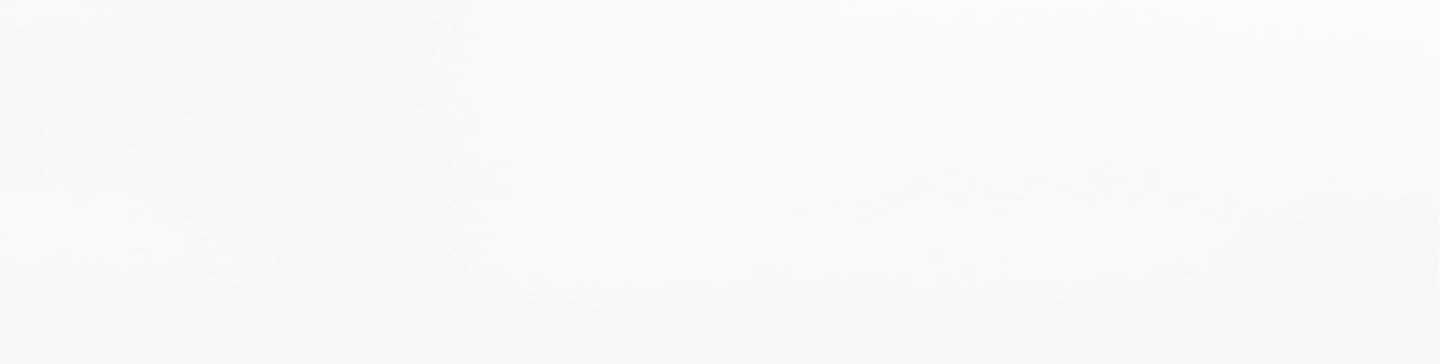
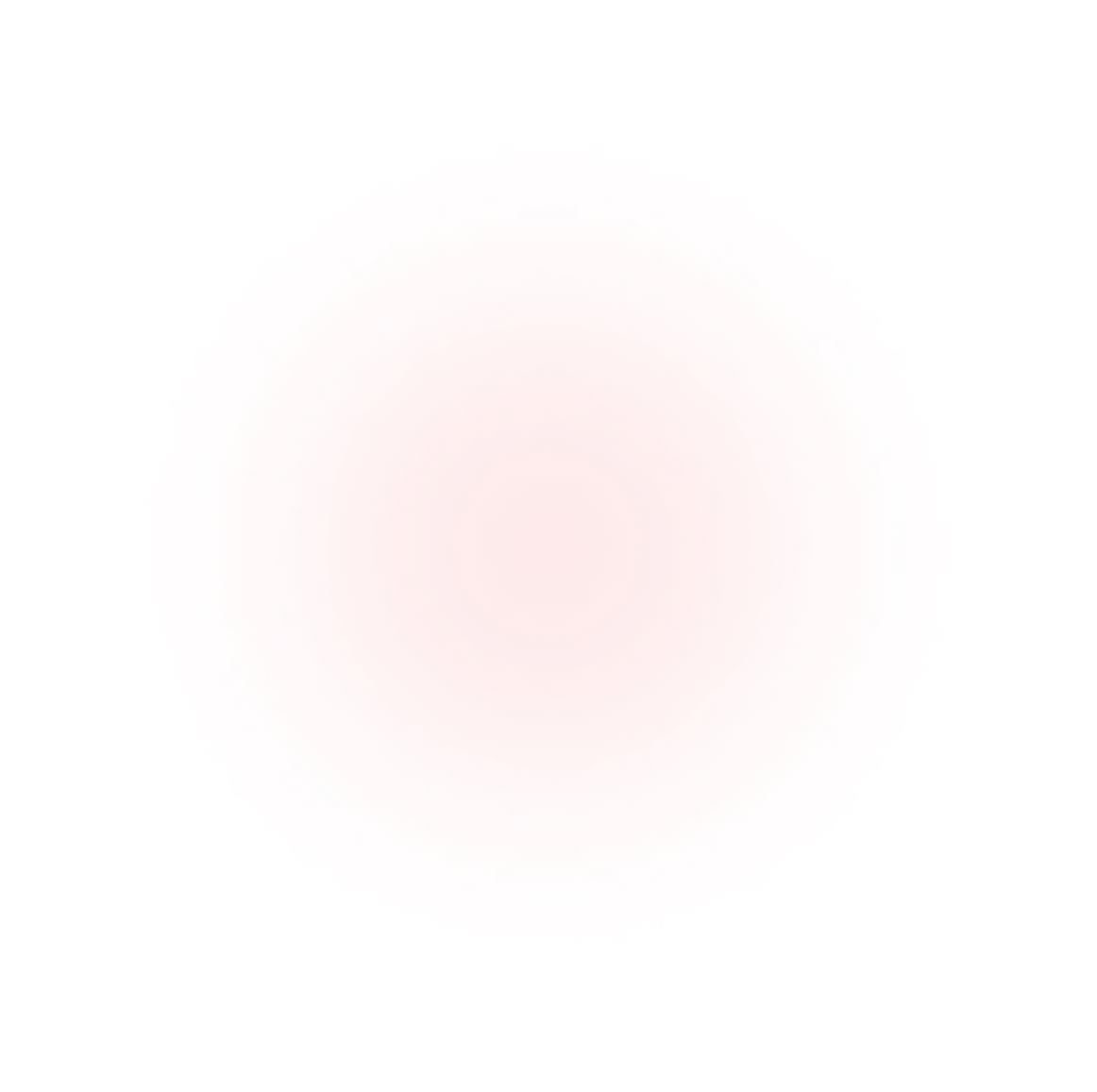
A Java Developer's Guide to Jedis on Windows with Memurai - the Windows-Native Port of Redis
Technical articles and news about Memurai.
Categories
You might be here because, despite trawling through stack overflow forums, Jedis is still playing mind tricks with your Windows servers. If that's the case, then worry no more, the force of Memurai is here to help! Designed for Windows environments, Memurai is a high-performance in-memory cache and persistent datastore, fully compatible with Redis 7.2.
In the first part of this blog, we’ll introduce you to the basics of setting up Jedis with Memurai, demonstrating its advantages over other solutions like Docker and WSL, and guiding you through creating a simple Java application for efficient data storage and retrieval.
In the second part of this blog, we'll elevate the discussion to encompass advanced features suited for production-grade deployments. Part 2 is ideal for professionals seeking to scale their Java applications and enhance reliability and efficiency on Windows platforms. We'll delve into sophisticated capabilities like connection pooling, high availability with Redis Sentinels, and explore scaling your deployments with Redis clusters. Let’s dive in!
What is Jedis?
Jedis - a clever portmanteau of Java and Redis - is a popular client library for interacting with Redis servers. It provides a Java API to connect, send commands, and retrieve data from a Redis server and allows developers to work with Redis in Java applications efficiently.
Comparing Memurai and Redis on Windows
While Redis is a popular choice for many developers, it's not supported on Windows. This means that running Redis on a Windows server will require using Docker, WSL, or outdated versions that can compromise security and add complexity. Memurai, on the other hand, offers seamless integration with Windows, making it a more straightforward and efficient choice. For a deeper comparison, check out our blogs on Running Redis with Docker and WSL/WSL2. Memurai is 100% compatible with the latest Redis protocol, so client libraries that works with Redis will also work with Memurai, including Jedis. Let's show you how to set up Jedis on Windows without hassle.
Installation and Setup
Before we dive into coding, it's essential to set up our environment. Memurai installation is straightforward and can be found in detail here. Once Memurai is up and running, let's focus on integrating Jedis, a popular Redis client library, with our Windows setup.
Setting Up Jedis:
• Ensure you have Java installed on your system.
• Include Jedis in your project's dependencies, typically via Maven or Gradle.
Part 1: Creating a Simple Java Application with Jedis and Memurai
We will create a basic application that stores and retrieves data using Jedis and Memurai. This real-world example will help you understand how to implement key-value storage operations.
Step 1: Initialize a Jedis Connection
import redis.clients.jedis.Jedis;
public class JedisExample {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost", 6379);
System.out.println("Connected to Memurai!");
// Further operations will go here.
}
}
This imports the necessary Jedis class and establishes a connection to a Memurai server running on the local machine (localhost) at the default Redis port (6379). When the connection is successful, it prints a message to the console indicating that the application is connected to Memurai.
Step 2: Basic Operations
// Setting a value
jedis.set("key", "value");
// Getting a value
String value = jedis.get("key");
System.out.println("Retrieved value: " + value);
This demonstrates how to perform basic key-value operations with Jedis. It shows how to use the set method to store a value associated with a key in Memurai and the get method to retrieve the value associated with a key. The retrieved value is then printed to the console.
Step 3: Error Handling and Best Practices
try {
// Operations
} catch (Exception e) {
e.printStackTrace();
} finally {
jedis.close();
}
Remember to handle exceptions and ensure proper resource management by closing the Jedis connection in a final block. This helps to prevent resource leaks and ensures that the application behaves correctly in the event of an error.
Practical Applications and Use Cases for Jedis on Windows Servers
The basic steps above serve as a starting point for developers looking to implement simple key-value storage operations using Jedis and Memurai/Redis on Windows. Combining Jedis and Memurai is ideal for a range of applications, from session caching in web applications to real-time analytics. The simplicity of our example can be expanded to suit more complex scenarios, such as managing shopping carts in e-commerce platforms or handling user sessions in large-scale applications.
Part 2: Advancing to Production-Grade Solutions with Memurai on Windows
So far, we've laid the groundwork for using Jedis with Memurai to create robust Java applications. But what about scenarios where your application demands go beyond the basics? In the next sections, we'll explore advanced features that elevate your application to meet production-grade standards in Windows environments.
In the upcoming sections, we'll explore sophisticated capabilities like connection pooling, Redis Sentinel support, and Redis cluster implementations. These topics are tailored for professionals aiming to elevate their Java applications in terms of scalability, reliability, and efficiency on Windows platforms.
Enhancing Performance with Connection Pools
In production environments, managing Memurai/Redis on Windows connections efficiently is crucial. This is where connection pools come into play. They ensure that a pre-established number of connections are ready to be used when needed, reducing the latency and load associated with establishing a connection.
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
public class RedisPool {
private static final JedisPool pool = new JedisPool(new JedisPoolConfig(), "localhost", 6379);
public static void main(String[] args) {
try (Jedis jedis = pool.getResource()) {
// Your code here
}
}
}
This code snippet sets up a JedisPool, which is a highly recommended practice for production-ready applications.
High Availability with Redis Sentinels on Windows
For those requiring high availability, Redis Sentinels are essential. They monitor your Memurai/Redis instances and provide automatic failover support. Jedis offers built-in Sentinel support, ensuring your Java application can maintain a stable connection to Memurai/Redis even when the primary server fails.
import redis.clients.jedis.JedisSentinelPool;
public class RedisSentinel {
public static void main(String[] args) {
Set<String> sentinels = new HashSet<>();
sentinels.add("localhost:26379"); // Sentinel address
try (JedisSentinelPool pool = new JedisSentinelPool("mymaster", sentinels)) {
// Your code here, using pool.getResource()
}
}
}
In this example, JedisSentinelPool is used to manage connections via Redis Sentinels.
Scaling Java Applications with Memurai and Redis Clusters on Windows
Redis clusters offer a way to scale out your Memurai deployment for those with growing data and performance needs. Jedis has the capability to work with Redis clusters by distributing data across multiple Memurai/Redis nodes, which can be highly appealing for those considering complex, high-performance setups.
import redis.clients.jedis.HostAndPort;
import redis.clients.jedis.JedisCluster;
public class RedisClusterExample {
public static void main(String[] args) {
Set<HostAndPort> nodes = new HashSet<>();
nodes.add(new HostAndPort("localhost", 7000));
try (JedisCluster jedisCluster = new JedisCluster(nodes)) {
// Your code here, using jedisCluster
}
}
}
This snippet demonstrates connecting to a Redis cluster using JedisCluster.
Conclusion
By following the steps outlined in this blog, you'll have a solid foundation for using Jedis with Memurai, starting from basic setup to advanced production-grade solutions. While we've kept this discussion concise, for those eager to dive deeper, Jedis documentation and tutorials, especially Baeldung's comprehensive guide, offer invaluable further insights and examples.
This exploration into the world of Memurai/Redis on Windows and Jedis is just the beginning of what you can achieve in enhancing your Java applications and system efficiencies. Dive into Memurai's free developer edition, a perfect starting point for new users. For production environments that demand the utmost in efficiency and reliability, reach out to us here to learn more about enhancing your data management capabilities with Memurai’s enterprise edition.
Thank you for reading, and happy coding!